index.asp
<html>
<head>
<title>Home Demo</title>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<STYLE> body,td,th { font-family: Arial, Helvetica, sans-serif; font-size: 12px; }
</STYLE>
<!-- THIS IS SCRIPT SECTION-->
<script language=javascript>
// load all need images before page will be shown in browser window
i1 = new Image; i1.src = "0.png";
i2 = new Image; i2.src = "1.png";
i3 = new Image; i3.src = "2.png";
i4 = new Image; i4.src = "3.png";
i5 = new Image; i5.src = "4.png";
i6 = new Image; i6.src = "5.png";
i7 = new Image; i7.src = "6.png";
i8 = new Image; i8.src = "7.png";
i9 = new Image; i9.src = "8.png";
i10 = new Image; i10.src = "9.png";
i11 = new Image; i11.src = "dot.png";
i12 = new Image; i12.src = "cel.png";
i13 = new Image; i13.src = "lux.png";
i14 = new Image; i14.src = "minus.png";
i15 = new Image; i15.src = "per.png";
i16 = new Image; i16.src = "digit.png";
i17 = new Image; i17.src = "empty.png";
i18 = new Image; i18.src = "occupied.png";
i19 = new Image; i19.src = "e1.png";
i20 = new Image; i20.src = "e2.png";
i21 = new Image; i21.src = "e3.png";
i22 = new Image; i22.src = "e4.png";
// Make request to the server each 30 seconds
// The response from the server update values
function AutoReload()
{
try
{
makeRequest(0, 'hall.asp');
makeRequest(1, 'reading.asp');
makeRequest(2, 'living.asp');
makeRequest(3, 'bedroom.asp');
makeRequest(4, 'dining.asp');
}
catch(e)
{
}
window.setTimeout("AutoReload()", 30000); // reinit timer
}
var n;
// Dynamic data request
var http_request = new Array(5);
http_request[0] = false;
http_request[1] = false;
http_request[2] = false;
http_request[3] = false;
http_request[4] = false;
function makeRequest(room, url) {
if( !http_request[room] )
{
if (window.XMLHttpRequest) { // Mozilla, Safari,...
http_request[room] = new XMLHttpRequest();
if (http_request[room].overrideMimeType) {
http_request[room].overrideMimeType('text/xml');
}
} else if (window.ActiveXObject) { // IE
try {
http_request[room] = new ActiveXObject("Msxml2.XMLHTTP");
} catch (e) {
try {
http_request[room] = new ActiveXObject("Microsoft.XMLHTTP");
} catch (e) {}
}
}
}
if (!http_request[room]) {
alert('Giving up :( Cannot create an XMLHTTP instance');
return false;
}
if(http_request[room].readyState == 0)
{
switch(room)
{
case 0 :
http_request[room].onreadystatechange = ShowResultHall;
break;
case 1 :
http_request[room].onreadystatechange = ShowResultReading;
break;
case 2 :
http_request[room].onreadystatechange = ShowResultLiving;
break;
case 3 :
http_request[room].onreadystatechange = ShowResultBedroom;
break;
case 4 :
http_request[room].onreadystatechange = ShowResultDining;
break;
default:
alert("Wrong number for room!");
}
http_request[room].open("GET", url, true);
http_request[room].send(null);
}
}
function ConvertHumidity(rh, Temperature)
{
var C1=-4.0;
var C2=0.0405;
var C3=-0.0000028;
var T1=0.01;
var T2=0.00008;
var t_C = Temperature *0.01 - 40;
var rh_lin=C3*rh*rh + C2*rh + C1;
var rh_true=(t_C-25)*(T1+T2*rh)+rh_lin;
if(rh_true>100){rh_true=100;}
if(rh_true<0.1){rh_true=0.1;}
return Math.round(rh_true*10)/10;
}
function ConvertTemperature(t)
{
return Math.round((t*0.01-40.0)*10)/10;
}
// get value from text parameter
function getValue(Text, Key)
{
var pos, prev, n;
var Line;
if( Text.length != 0 )
{
pos = 0; prev = 0;
while ( pos != -1 )
{
pos = Text.indexOf(';', pos);
if( pos != -1 )
{
Line = Text.substring(prev, pos);
n = Line.indexOf(':');
if( n == -1)
{
alert('There was a problem with the response text.');
}
if( Key == Line.substring(0, n) )
{
return Line.substring(n+1, Line.length);
}
pos = pos + 1;
prev = pos;
}
}
}
}
// update Activity value
function ParseActivity(Room, Activity)
{
var name = Room + "Act";
var Image = document.images(name);
Activity = Activity * 1;
if (Activity == 0)
Image.src = "empty.png";
else
Image.src = "occupied.png";
}
// update temperature value
function ParseTemperature(Room, Temperature)
{
var num, i, k, n, t;
if( Temperature == 65535 )
{
n = 4
while( n > 0 )
{
var name = Room + "T" + n;
var Image = document.images(name);
Image.src = "e" + n + ".png";
n = n - 1;
}
return;
}
t = ConvertTemperature(Temperature);
t = t.toString(10);
i = t.length;
k = i;
n = 4;
while( i != 0 )
{
var name = Room + "T" + n;
var Image = document.images(name);
num = t.substring(k-1, k)
if( num == ',' || num == '.')
num = "dot";
if( num == '-' )
num = "minus";
Image.src = num + ".png";
n = n - 1;
i = i - 1;
k = k - 1;
}
while( n > 0 )
{
var name = Room + "T" + n;
var Image = document.images(name);
Image.src = "digit.png";
n = n - 1;
}
}
// update light value
function ParseLight(Room, Light)
{
var num, i, k, n;
Light = Light * 1;
Light = Light.toString(10);
i = Light.length;
k = i;
n = 4;
while( i != 0)
{
var name = Room + "L" + n;
var Image = document.images(name);
num = Light.substring(k-1, k)
if( num == ',' || num == '.')
num = "dot";
Image.src = num + ".png";
n = n - 1;
i = i - 1;
k = k - 1;
}
while( n > 0 )
{
var name = Room + "L" + n;
var Image = document.images(name);
Image.src = "digit.png";
n = n - 1;
}
}
// update humidity value
function ParseHumidity(Room, Humidity, Temp)
{
var num, i, k, n, h;
if( Humidity == 65535 )
{
n = 4
while( n > 0 )
{
var name = Room + "H" + n;
var Image = document.images(name);
Image.src = "e" + n + ".png";
n = n - 1;
}
return;
}
h = ConvertHumidity(Humidity, Temp);
h = h.toString(10);
i = h.length;
k = i;
n = 4;
while( i != 0 )
{
var name = Room + "H" + n;
var Image = document.images(name);
num = h.substring(k-1, k)
if( num == ',' || num == '.')
num = "dot";
if( num == '-' )
num = "minus";
Image.src = num + ".png";
n = n - 1;
i = i - 1;
k = k - 1;
}
while( n > 0 )
{
var name = Room + "H" + n;
var Image = document.images(name);
Image.src = "digit.png";
n = n - 1;
}
}
//==========================================================================
// update all values in HALL section
function ShowResultHall()
{
if (http_request[0].readyState == 4)
{
if (http_request[0].status == 200)
{
// Parse response
ParseActivity("H", getValue(http_request[0].responseText, "Activity"));
}
http_request[0].abort();
}
}
//==========================================================================
// update all values in READINGROOM section
function ShowResultReading()
{
if (http_request[1].readyState == 4)
{
if (http_request[1].status == 200)
{
// Parse response
ParseLight("R", getValue(http_request[1].responseText, "Light"));
ParseTemperature("R", getValue(http_request[1].responseText, "Temperature"));
ParseHumidity("R", getValue(http_request[1].responseText, "Humidity"), getValue(http_request[1].responseText, "Temperature"));
ParseActivity("R", getValue(http_request[1].responseText, "Activity"));
}
http_request[1].abort();
}
}
//==========================================================================
// update all values in LIVINGROOM section
function ShowResultLiving()
{
if (http_request[2].readyState == 4)
{
if (http_request[2].status == 200)
{
// Parse response
ParseLight("L", getValue(http_request[2].responseText, "Light"));
ParseTemperature("L", getValue(http_request[2].responseText, "Temperature"));
ParseHumidity("L", getValue(http_request[2].responseText, "Humidity"), getValue(http_request[2].responseText, "Temperature"));
ParseActivity("L", getValue(http_request[2].responseText, "Activity"));
}
http_request[2].abort();
}
}
//==========================================================================
// update all values in BADROOM section
function ShowResultBedroom()
{
if (http_request[3].readyState == 4)
{
if (http_request[3].status == 200)
{
// Parse response
ParseLight("B", getValue(http_request[3].responseText, "Light"));
ParseTemperature("B", getValue(http_request[3].responseText, "Temperature"));
ParseHumidity("B", getValue(http_request[3].responseText, "Humidity"), getValue(http_request[3].responseText, "Temperature"));
ParseActivity("B", getValue(http_request[3].responseText, "Activity"));
}
http_request[3].abort();
}
}
//==========================================================================
// update all values in DININGROOM section
function ShowResultDining()
{
if (http_request[4].readyState == 4)
{
if (http_request[4].status == 200)
{
// Parse response
ParseLight("D", getValue(http_request[4].responseText, "Light"));
ParseTemperature("D", getValue(http_request[4].responseText, "Temperature"));
ParseHumidity("D", getValue(http_request[4].responseText, "Humidity"), getValue(http_request[4].responseText, "Temperature"));
ParseActivity("D", getValue(http_request[4].responseText, "Activity"));
}
http_request[4].abort();
}
}
</script>
</head>
<body bgcolor="white" onload="AutoReload();">
<TABLE WIDTH="100%" HEIGHT="100%">
<!-- THIS SECTION IMPLEMENTS WEBCAT LOGO IMAGE AT THE TOP OF THE PAGE -->
<tr HEIGHT="1%">
<TD><img src="banner.gif" width="349" height="90" border="0"></TD>
</tr>
<TR>
<TD ALIGN=center VALIGN=middle>
<h3><font color=gray face="Tahoma; Arial" size="2">WEBCAT Status Page</font></h3>
<h3>Floor Plan</h3>
<!-- FOLLOW TABLE PRESENT HOUSE PLAN IMAGE ON THE PAGE-->
<table cellpadding=0 cellspacing=0 background="digit.png" border=0 width=800>
<tr>
<td colspan=7 width=822><img src="top.png" width=822 height=64 border=0 vspace=0 hspace=0></td>
</tr>
<tr>
<td width=195><img src="10.png" width=195 height=180 border=0 vspace=0 hspace=0></td>
<td width=110 align=left valign=top>
<!-- FOLLOW SECTION PRESENT READING ROOM -->
<table border=0 cellpadding=0 cellspacing=0 height=180 width="100%">
<tr>
<td align=right>
<img id="RL1" src="digit.png" hspace=0 vspace=0>
<img id="RL2" src="digit.png" hspace=0 vspace=0>
<img id="RL3" src="digit.png" hspace=0 vspace=0>
<img id="RL4" src="digit.png" hspace=0 vspace=0>
<img src="lux.png">
</td>
</tr>
<tr>
<td align=right>
<img id="RAct" src="digit.png" hspace=0 vspace=0>
</td>
</tr>
<tr>
<td align=right>
<img id="RT1" src="digit.png" hspace=0 vspace=0>
<img id="RT2" src="digit.png" hspace=0 vspace=0>
<img id="RT3" src="digit.png" hspace=0 vspace=0>
<img id="RT4" src="digit.png" hspace=0 vspace=0>
<img src="cel.png">
</td>
</tr>
<tr>
<td align=right>
<img id="RH1" src="digit.png" hspace=0 vspace=0>
<img id="RH2" src="digit.png" hspace=0 vspace=0>
<img id="RH3" src="digit.png" hspace=0 vspace=0>
<img id="RH4" src="digit.png" hspace=0 vspace=0>
<img src="per.png">
</td>
</tr>
</table>
<!-- HERE IS THE END OF READINGROOM SECTION -->
</td>
<td width=339 colspan=3><img src="11.png" width=339 height=180 border=0 vspace=0 hspace=0></td>
<td width=110 align=left valign=top>
<!-- FOLLOW SECTION PRESENT LIVING ROOM -->
<table border=0 cellpadding=0 cellspacing=0 height=180 width="100%" id="tb2" style='FILTER: revealTrans(duration=1,transition=5)'>
<tr>
<td align=right>
<img id="LL1" src="digit.png" hspace=0 vspace=0>
<img id="LL2" src="digit.png" hspace=0 vspace=0>
<img id="LL3" src="digit.png" hspace=0 vspace=0>
<img id="LL4" src="digit.png" hspace=0 vspace=0>
<img src="lux.png">
</td>
</tr>
<tr>
<td align=right>
<img id="LAct" src="digit.png" hspace=0 vspace=0>
</td>
</tr>
<tr>
<td align=right>
<img id="LT1" src="digit.png" hspace=0 vspace=0>
<img id="LT2" src="digit.png" hspace=0 vspace=0>
<img id="LT3" src="digit.png" hspace=0 vspace=0>
<img id="LT4" src="digit.png" hspace=0 vspace=0>
<img src="cel.png">
</td>
</tr>
<tr>
<td align=right>
<img id="LH1" src="digit.png" hspace=0 vspace=0>
<img id="LH2" src="digit.png" hspace=0 vspace=0>
<img id="LH3" src="digit.png" hspace=0 vspace=0>
<img id="LH4" src="digit.png" hspace=0 vspace=0>
<img src="per.png">
</td>
</tr>
</table>
<!-- HERE IS THE END OF LIVINGROOM SECTION -->
</td>
<td width=68><img src="12.png" width=68 height=180 border=0 vspace=0></td>
</tr>
<tr>
<td colspan=8 width=822><img src="middle.png" width=822 height=68 border=0 vspace=0 hspace=0></td>
</tr>
<tr>
<td width=195 rowspan=2><img src="20.png" width=195 height=180 border=0 vspace=0 hspace=0></td>
<td width=110 align=left valign=top rowspan=2>
<!-- FOLLOW SECTION PRESENT BADROOM -->
<table border=0 cellpadding=0 cellspacing=0 height=180 width="100%" id="tb3" style='FILTER: revealTrans(duration=1,transition=5)'>
<tr>
<td align=right>
<img id="BL1" src="digit.png" hspace=0 vspace=0>
<img id="BL2" src="digit.png" hspace=0 vspace=0>
<img id="BL3" src="digit.png" hspace=0 vspace=0>
<img id="BL4" src="digit.png" hspace=0 vspace=0>
<img src="lux.png">
</td>
</tr>
<tr>
<td align=right>
<img id="BAct" src="digit.png" hspace=0 vspace=0>
</td>
</tr>
<tr>
<td align=right>
<img id="BT1" src="digit.png" hspace=0 vspace=0>
<img id="BT2" src="digit.png" hspace=0 vspace=0>
<img id="BT3" src="digit.png" hspace=0 vspace=0>
<img id="BT4" src="digit.png" hspace=0 vspace=0>
<img src="cel.png">
</td>
</tr>
<tr>
<td align=right>
<img id="BH1" src="digit.png" hspace=0 vspace=0>
<img id="BH2" src="digit.png" hspace=0 vspace=0>
<img id="BH3" src="digit.png" hspace=0 vspace=0>
<img id="BH4" src="digit.png" hspace=0 vspace=0>
<img src="per.png">
</td>
</tr>
</table>
<!-- HERE IS THE END OF BADROOM SECTION -->
</td>
<td width=68><img src="21.png" width=68 height=57 border=0 vspace=0 hspace=0></td>
<td width=80 align=left valign=top>
<!-- FOLLOW SECTION PRESENT HALL -->
<table border=0 cellpadding=0 cellspacing=0 width="100%" id="tb4" style='FILTER: revealTrans(duration=1,transition=5)'>
<tr>
<td align=center>
<img id="HAct" src="digit.png" hspace=0 vspace=0 border=0>
</td>
</tr>
</table>
<!-- HERE IS THE END OF HALL SECTION -->
</td>
<td width=191><img src="22.png" width=191 height=57 border=0 vspace=0 hspace=0></td>
<td width=110 align=left valign=top rowspan=2>
<!-- FOLLOW SECTION PRESENT DININGROOM -->
<table border=0 cellpadding=0 cellspacing=0 height=180 width="100%" id="tb5" style='FILTER: revealTrans(duration=1,transition=5)'>
<tr>
<td align=right>
<img id="DL1" src="digit.png" hspace=0 vspace=0>
<img id="DL2" src="digit.png" hspace=0 vspace=0>
<img id="DL3" src="digit.png" hspace=0 vspace=0>
<img id="DL4" src="digit.png" hspace=0 vspace=0>
<img src="lux.png">
</td>
</tr>
<tr>
<td align=right>
<img id="DAct" src="digit.png" hspace=0 vspace=0>
</td>
</tr>
<tr>
<td align=right>
<img id="DT1" src="digit.png" hspace=0 vspace=0>
<img id="DT2" src="digit.png" hspace=0 vspace=0>
<img id="DT3" src="digit.png" hspace=0 vspace=0>
<img id="DT4" src="digit.png" hspace=0 vspace=0>
<img src="cel.png">
</td>
</tr>
<tr>
<td align=right>
<img id="DH1" src="digit.png" hspace=0 vspace=0>
<img id="DH2" src="digit.png" hspace=0 vspace=0>
<img id="DH3" src="digit.png" hspace=0 vspace=0>
<img id="DH4" src="digit.png" hspace=0 vspace=0>
<img src="per.png">
</td>
</tr>
</table>
<!-- HERE IS THE END OF DININGROOM SECTION -->
</td>
<td width=68 rowspan=2><img src="23.png" width=68 height=180 border=0 vspace=0 hspace=0></td>
</tr>
<tr>
<td width=339 colspan=3><img src="220.png" width=339 height=123 border=0 vspace=0 hspace=0></td>
</tr>
<tr>
<td colspan=7 width=822><img src="bottom.png" width=822 height=80 border=0 vspace=0 hspace=0></td>
</tr>
</table>
</TD>
</TR>
<tr height="1%">
<td>
<HR>
<p align=right><font color=gray face="Tahoma; Arial" size="2">Copyright ?1998-2005 BiPOM Electronics </font></p>
</td>
</tr>
</TABLE>
</body>
</html>
hall.asp
Activity:^P1.0^;
reading.asp
Temperature:^SHT7XTEMP^;Humidity:^SHT7XHUMI^;Activity:^P1.0^;Light:^P1.1^;
living.asp
Temperature:^SHT7XTEMP^;Humidity:^SHT7XHUMI^;Activity:^P1.0^;Light:^P1.1^;
bedroom.asp
Temperature:^SHT7XTEMP^;Humidity:^SHT7XHUMI^;Activity:^P1.0^;Light:^P1.1^;
dining.asp
Temperature:^SHT7XTEMP^;Humidity:^SHT7XHUMI^;Activity:^P1.0^;Light:^P1.1^;
Screenshot
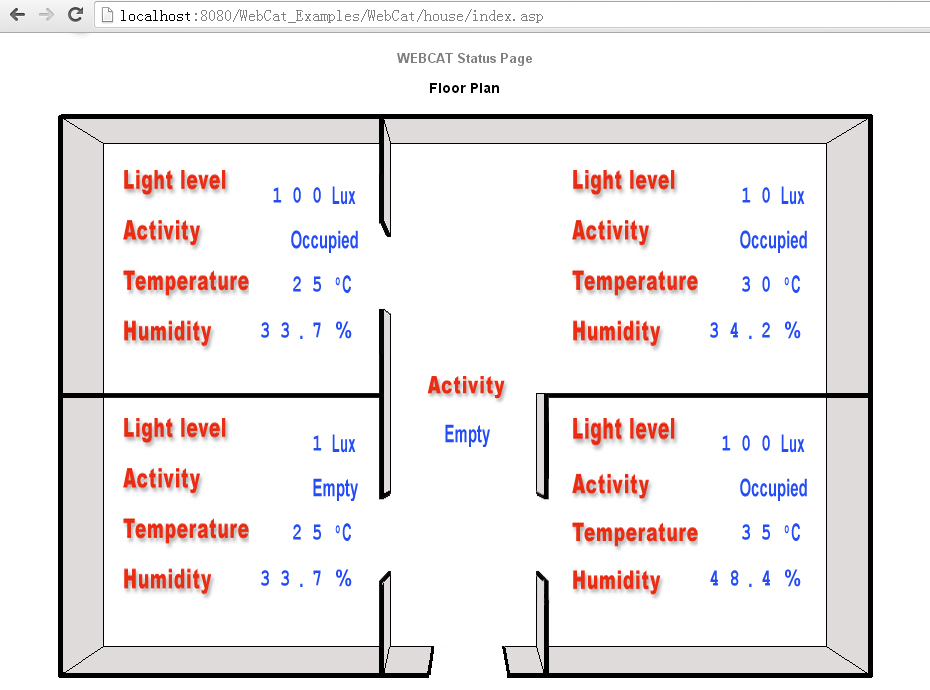